일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 프리온보딩
- state
- 원티드
- Reducer
- Frontend
- axios
- CORS
- es6
- JavaScript
- 컴포넌트
- v9
- TypeScript
- array
- 타입스크립트
- 리액트
- 알고리즘
- Redux
- 자바스크립트
- til
- react localStorage
- JS
- 파이어베이스
- Component
- firebase
- 브라우저
- react
- localstorage
- 프론트엔드
- 비트 연산자
- 프로그래머스
- Today
- Total
도리쓰에러쓰
[React] ref를 prop으로 넘기기 - forwardRef() 본문
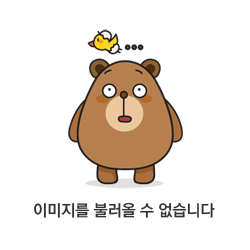
🔽 우선 forwardRef()을 알기 전에 ref를 알아야 하는데 아래 게시물을 참고하면 좋을 것 같다.
[React] Ref 사용하여 DOM 요소에 접근하기 - useRef()
1. Ref란? React에서 DOM에 직접 접근하기 위해 사용한다. HTML로 예를 들자면, 에 접근하고 싶을 때 "div-id"라는 id를 사용하여 DOM 요소에 접근한다. HTML을 작성할 때 id로 DOM 요소를 접근하는 것처럼, Rea
dori-coding.tistory.com
1. forwardRef()란?
React에서 일반적으로 사용할 수 없는 prop이 몇 가지 있다. 그 중 ref prop도 HTML Element 접근이라는 특수한 용도로 사용되기 때문에 일반적인 prop으로 사용할 수 없다.
React Component에서 ref prop을 사용하려면 forwardRef()라는 함수를 사용해야 한다. React Component를 forwardRef() 함수로 감싸주면, 컴포넌트 함수는 2번째 매개변수를 갖게 되는데 이를 통해 ref prop을 넘길 수 있다.
2. forwardRef() 사용하기
우선 forwardRef()를 사용하지 않은 아래 예시를 보자.
import { useRef } from "react";
const Input = ref => {
return <input type="text" ref={ref} />;
};
const Field = () => {
const inputRef = useRef(null);
const focusHandler = () => {
inputRef.current.foucus();
};
return(
<>
<Input ref={inputRef} />
<button onClick={focusHandler}>focus</button>
</>
);
};
<Field /> Component에 useRef() 함수로 생성한 inputRef 객체가 있다. 이 객체를 자식인 <Input /> Component에 ref prop으로 넘긴다. 그러면 자식 <Input /> Component는 ref prop으로 넘어온 inputRef 객체를 다시 내부에 있는 <input> Element의 ref prop으로 넘겨준다.
하지만 위 예시에서는 ref를 prop으로 넘겨줬기 때문에 경고 메세지가 뜬다.
Warning: Input: `ref` is not a prop. Trying to access it will result in `undefined` being returned. If you need to access the same value within the child component, you should pass it as a different prop. (https://reactjs.org/link/special-props)
이제 forwardRef()를 적용해보자.
import { useRef, forwardRef } from "react";
const Input = forwardRef((props, ref) => {
return <input type="text" ref={ref} />;
});
const Field = () => {
const inputRef = useRef(null);
const focusHandler = () => {
inputRef.current.foucus();
};
return(
<>
<Input ref={inputRef} />
<button onClick={focusHandler}>focus</button>
</>
);
};
<Input /> Component를 forwardRef()로 감싸주고, 2번째 매개변수로 ref를 받아 <input> Element에 ref prop을 넘겼더니 경고 메세지 없이 버튼을 클릭하면 input에 포커스가 된 것을 확인할 수 있다.
'React > React' 카테고리의 다른 글
[React] 라이프사이클(Lifecycle)에 대해 (0) | 2022.07.13 |
---|---|
[React] useCallback()과 useMemo() 사용하기 (0) | 2022.05.01 |
[React] 전역 상태 관리하기 - useContext() (0) | 2022.04.21 |
[React] Ref 사용하여 DOM 요소에 접근하기 - useRef() (0) | 2022.04.21 |
[React] 제어 컴포넌트(Controlled Component)와 비제어 컴포넌트(Uncontrolled Component) (1) | 2022.04.08 |